In Solidity, there are types like value types (Value Types), reference types (Reference Types), and mapping types (Mapping Types). Value types are used to define numerical and string types. In value types, the values are stored directly (the difference from reference types will be mentioned on day 9).
Boolean Type
The bool (boolean type) is a data type that represents true or false values. It can take two values: “true” or “false”. For example, the variable isSuccessful, which indicates whether something is successful or not, is initially false, but can be set to true if things go well later.
bool isSuccessful = false;
The following operators are used to calculate true or false (assuming variables a and b are both bool type, with a=true and b=false):
- ! … Negation
- !a … If a is true, the opposite is false.
- && … AND condition
- a && b … Becomes true if both are true or both are false, so here it is false.
- || … OR condition
- a || b … Becomes true if either is true, so here it is true.
- == … Equality (whether they are the same)
- a == b … Becomes true if a and b are the same value, so here it is false.
- != … Inequality (whether they are not the same)
- a != b … Becomes true if a and b are different values, so here it is true.
Integer Types
The ‘int’ data type represents integers, and ‘uint’ represents positive integers. There are definitions ranging from int8 to int256 and uint8 to uint256, where the numbers following int and uint indicate the number of bits. The largest numbers, such as uint256, are the most commonly used. uint256 is an integer type that can handle very large numbers, up to 2^256 – 1. The following example defines a variable representing the amount of ETH. 1e18 is equivalent to 1000000000000000000, meaning the variable etherAmount represents 1000000000000000000 Wei (for currency units, refer to Day 4).
uint256 etherAmount = 1e18;
Address Type
The ‘address’ data type represents an Ethereum address and holds a 20-byte value. To see an example of an address type value, it’s a good idea to open MetaMask. Looking at MetaMask, you will see a string starting with 0x under the ‘AccountN’. This is the value of the address, and you can copy it using the icon on the right.
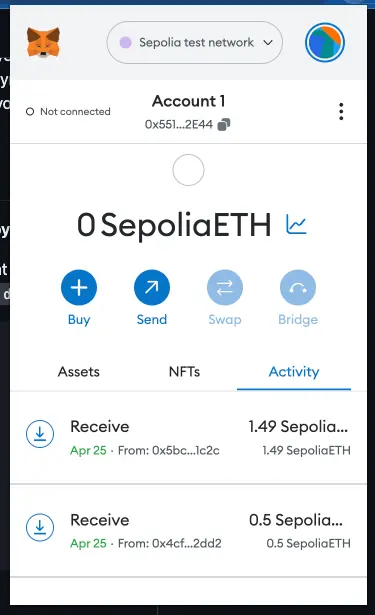
The value of the address in the above figure (for a test account) is “0x551af3f6A226a62BCb27f08B42401bd5629f2E44”. The initial “0x” indicates that it is in hexadecimal. A single digit in hexadecimal ranges from 0 to 15, using 0 to 9 and a to f in display. This corresponds to 4 bits (16 varieties). 551 to E44 totals 40 characters. Therefore, 4 bits x 40 characters = 160 bits = 20 bytes.
address public owner = 0x551af3f6A226a62BCb27f08B42401bd5629f2E44;
Byte Type
There are two types of byte arrays: fixed-length byte arrays from bytes1 to bytes32, ranging from 1 to 32 bytes, and the variable-length byte array ‘bytes’. Fixed-length means the length is constant, for example, bytes32 is always 32 bytes long. In contrast, variable-length means the length can change. Both store 1 byte (8 bits) of data per element.
Byte arrays are a convenient data type for efficiently storing and manipulating binary data. Text, images, and other data can be stored in byte arrays. For example, the string “cat” can be represented as a byte array like 0x123d33af44343. Byte arrays are used for various purposes, such as storing the contents of files in binary format.
function callSomething public {
...
bytes memory someBytes; // Byte array
...
}
String Type
The string type, as the name suggests, is used for handling text. However, as mentioned earlier, strings are stored as byte arrays, so their actual form is bytes. They are UTF-8 encoded strings (strictly speaking, not a value type).
string public uri = "https://token-village.com";
Enumeration Type
‘enum’ is a data type used for choosing from a predefined set of values. The following State variable has three values. In reality, these are represented as numbers: OPEN is 0, CLOSED is 1, and CANCELD is 2.
enum State {
OPEN,
CLOSED,
CANCELED
}
Using enums allows you to write clear and readable code when specifying certain values. For example, enums can be used as the type of function arguments or variables.
Additionally, since enums are treated as integer values, you can compare enum values numerically and use arithmetic operators with them.
Comments