In this guide, we will explain the configuration and process flow of the frontend for Web3, as well as an overview of the main technologies used.
Logical Architecture Diagram
The logical architecture from the frontend to the blockchain can be illustrated as follows:
- Local (user device): The environment where a browser and digital wallets like Metamask operate (Metamask is assumed here).
- Frontend
- Content Hosting Service: A service for displaying static sites (examples include S3, Netlify, Vercel, but here we assume IPFS).
- Web Server: An environment where node.js operates (an application built on Next.js running on node.js).
- Blockchain Network: The blockchain network where contracts are deployed.
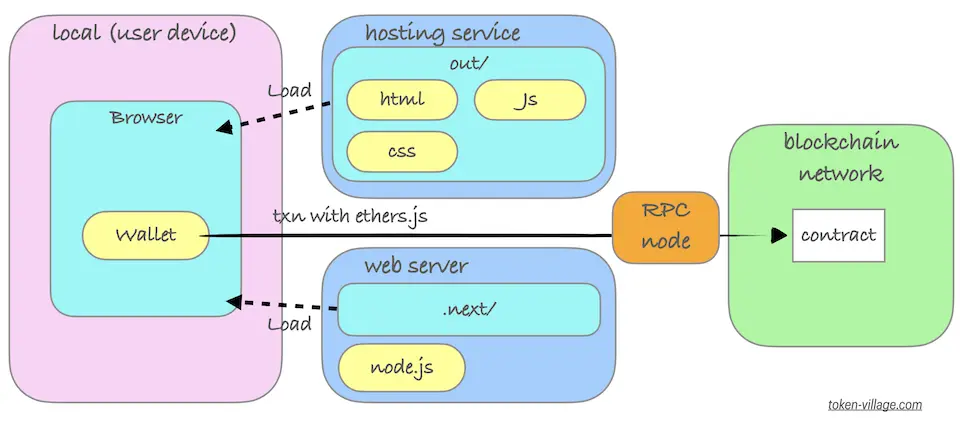
Process Flow
The process flow from the local terminal to the reception of a transaction by a contract deployed on the blockchain network is as follows:
- Loading the Static Site: Users access a Web3-enabled website hosted statically via a web browser. HTML, CSS, JavaScript files, etc., are downloaded from the hosting service and rendered on the local browser.
- Operations on the Browser: Users perform some action (e.g., clicking a button to make a transfer), and this action generates a blockchain transaction through libraries like web3.js or ethers.js.
- Transmission to RPC Node: To communicate with the blockchain network, the generated transaction is sent to an RPC (Remote Procedure Call) node (examples include Infura, Alchemy, or a proprietary Ethereum node).
- Transmission to the Blockchain: The RPC node forwards the transaction to the blockchain network (to a specific smart contract or address).
- Transaction Confirmation and Result Retrieval: After the transaction is included in a block and confirmed, its result (success or failure) is returned to the application via the RPC node. The application uses this information, for example, to display the transaction result to the user.
※ This flow assumes the use of a content hosting service. In the case of server-side rendering (discussed later), it is loaded from a web server running Node.js.
Technologies Used
Here, we explain an overview of the main technologies that will be used in the following development.
React
React is a JavaScript library for building user interfaces (UI).
Component-Based
UI is divided into reusable components. The following code is an example of a component named Greeting and how it is used elsewhere.
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
// Example usage:
<Greeting name="John" />
Declarative
It describes declaratively how data affects the UI. When data changes, React automatically manages which parts to update. The following code is an example of state. Each time it’s clicked, setCount is called, updating the count value, and the UI is automatically updated.
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
React Hooks
A new API that allows handling state and lifecycle events within functional components. useState and useEffect in the following code example are both part of React hooks.
Though overlapping with the previous point, useState is a Hook for “state management.” State management means monitoring a variable and re-rendering when the variable’s value is updated.
- initialValue: The initial value of the state
- state: The current value of the state
- setState: A function to update the state
useEffect is a Hook for handling “side effects.” A side effect is a specific operation performed when the value of a monitored variable changes. In the code example, it monitors the count variable and, when its value changes, executes document.title = Count: ${count}
(changing the title). The [count]
part here is called the dependency array.
import { useState, useEffect } from "react";
function App() {
const [state, setState] = useState(initialValue);
useEffect(() => {
document.title = `Count: ${count}`;
}, [count]); // By specifying "count" in the dependency array, this side effect will only be executed when the state of "count" changes.
return <div>{data}</div>;
}
Server Side Rendering
For SEO and performance improvement, it is also possible to generate HTML on the server side. Server-side rendering is primarily implemented using frameworks like Next.js.
Next.js
Next.js is a framework based on React (primarily for frontend, but it can also be used for backend implementation with simple logic). It has the following characteristics:
- Hybrid Rendering: Next.js is a hybrid framework that allows the use of Server-Side Rendering (SSR), Static Site Generation (SSG), and Client-Side Rendering (CSR). This flexibility enables optimization of performance, SEO, and user experience.
- Zero Configuration: Next.js follows a philosophy of “no configuration needed.” It automatically handles initial setup, routing, and Webpack configurations with minimal requirements.
- Optimized Performance: Next.js features code splitting, lazy loading, and prefetching for optimization, enabling fast page loading and transitions.
- File-Based Routing: It automatically generates frontend routing based on the file structure in the mainly used pages directory.
- API Routes: Part of the routing functionality in point 4, using the pages/api directory allows for easy creation of API endpoints. This integrates frontend and backend code within the same project.
These features offer advantages when using Next.js in combination with React, compared to using React alone. In fact, it is recommended to use frameworks like Next.js or Remix in conjunction with React.
React-Moralis
react-moralis
is a library for easy use of Moralis within React applications. Moralis is a platform that simplifies the development of blockchain applications and reduces the need for backend setup and management. Specifically, it provides common backend functionalities like user authentication, data storage, real-time data, and chain data queries.
react-moralis
provides React Hooks and providers for intuitively using Moralis’s features in React applications.
In the example of this tutorial, Moralis’s services are not used directly; rather, the focus is on its use as a library for the frontend. Specifically, hooks provided by Moralis, such as useMoralis and useWeb3Contract, are used. These are utilized for fetching chain or account information and calling transactions for deployed contracts from the frontend.
Comments