In coding, error handling is a crucial element. Creating code that returns clear errors at the appropriate time helps in analyzing problems and deriving improvement measures when issues occur. This guide explains error handling in Solidity.
revert
The revert
function is used to discard all changes attempted up to that point and return to the original state. Typically, it is used in situations where, if a certain undesirable condition is met, the function reverts with an error message.
When executing revert, you can either include an error message as an argument or attach a predefined custom error type.
The following example shows a modification of the fund
function from Day 11, where it reverts with an error under certain conditions.
The first revert is executed with a custom error, which is defined outside of the contract. Custom errors can be defined to take arguments like this.
The second revert is executed with an error message as an argument.
// SPDX-License-Identifier: MIT
pragma solidity 0.8.13;
error Fund__NotEnoughETH(uint256 fundedAmount); // Custom error
contract Funding {
...
function fund() public payable {
if (msg.value < 100000000000000000) {
revert Fund__NotEnoughETH(msg.value); // if the amount is less than 0.1ETH, revert with a customer error
} else if (msg.value > 10000000000000000000) {
revert("Funded amount should be less than 10 ETH!"); // revert with an error message as an argument
}
s_funders.push(msg.sender);
s_addressToAmount[msg.sender] += msg.value;
}
...
When each condition is met, errors are displayed on the Remix console as shown in the respective images. With custom errors, specific values (like how much was attempted to be funded and failed) can also be checked.
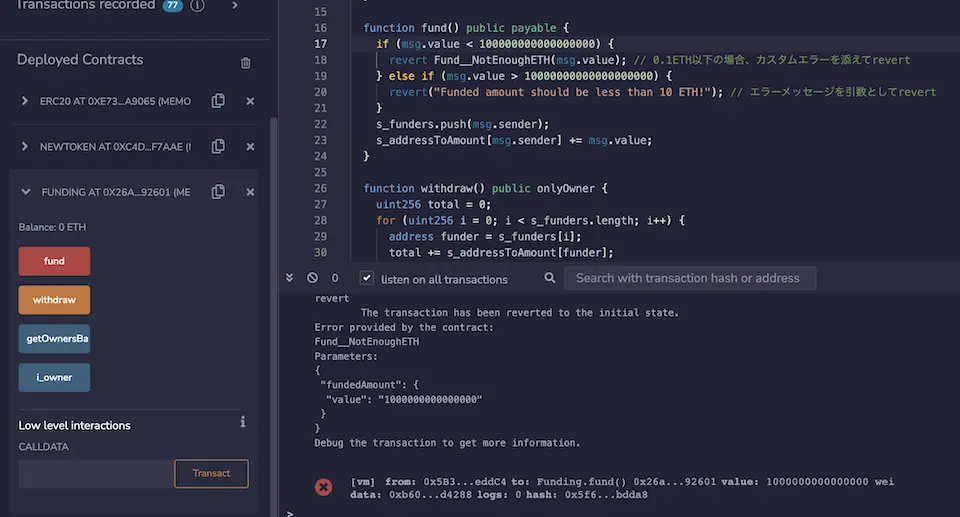
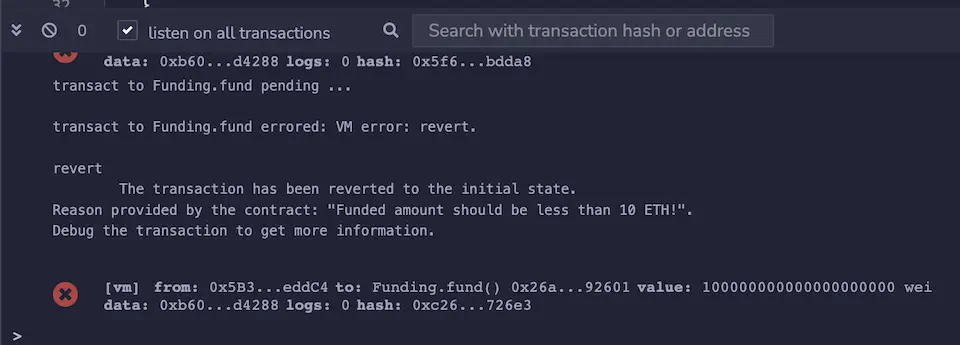
require
require
is a function used for error checking and condition verification. It takes a condition and an error message as arguments. If the condition is met, the process continues; if not, an error message is output, and the process is halted.
The following example adds a condition to the fund
function from the same Day 11. If the amount of Ether sent is more than 0.1 ETH, lines 4 and 5 are executed. If it’s less than or equal to 0.1 ETH, a process error occurs, and the transaction is reverted.
...
function fund() public payable {
require(msg.value > 100000000000000000, "value should be greater than 0.1 ETH");
s_funders.push(msg.sender);
s_addressToAmount[msg.sender] += msg.value;
}
...
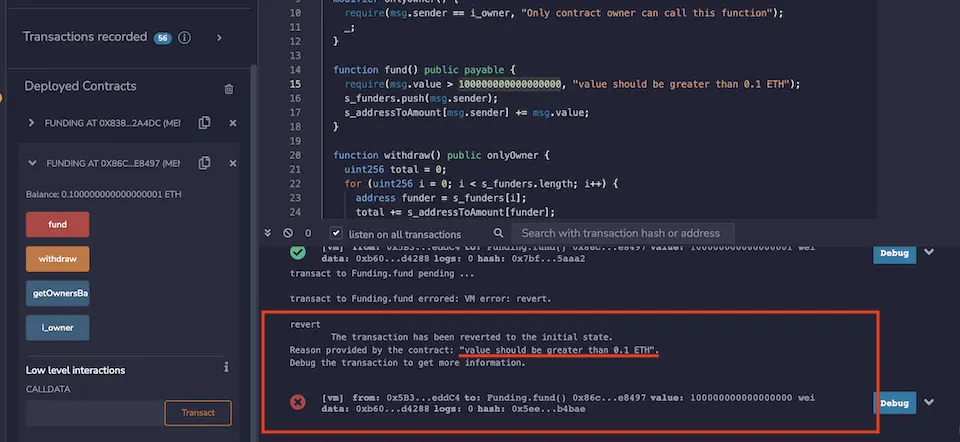
Comments