In Solidity, events are a mechanism for notifying the external world. Using events, external entities (such as frontend applications) can receive information from triggered events and perform various actions.
Workflow
As mentioned at the beginning, events are used to notify external applications of certain occurrences (which are monitored to be informed of these events). The process of handling events follows this flow:
- A transaction occurs, executing functions or other processes.
- An event is triggered (
emit
). - External applications listen for (monitor) these triggered events.
The frontend application performs operations based on the received event information, or the information is saved to a database*. Then, the frontend application queries this database to display the received information.
* This refers to a database outside of the blockchain, often termed “off-chain.”
Events in Solidity are stored in the transaction logs (log area) of the blockchain. These logs serve as a record of blockchain events, containing relevant data at the time of the event’s firing. This separation of event data from the contract’s state helps reduce storage costs.
Event/Emit
Next, let’s look at how to specifically define events. There are two main parts: defining the event itself and defining when and under what conditions the event is triggered (emitted).
Here is an example of event definition. An event can have parameters, which external applications can use when they receive these parameters along with the event. The syntax is event <EventName>(<Type> parameterName, ...);
.
event Funded(address indexed funder, uint256 indexed fundedAmount);
Events can have two types of parameters: indexed and non-indexed. The distinction affects how easily events can be searched. The former makes querying easier. It’s helpful to think of it like the index in a relational database.
※ Information about triggered events can be seen, for example, in the logs section on Etherscan.
An actual example of an event on Etherscan, involving the above-mentioned Funded event in the Funding contract, can be seen in the following image. This is the log of the event that was triggered after the fund function was called following the deployment of the Funding contract.
- Address: The contract address
- Name: The name of the function that triggered the event
- Topics: indexed parameters
- Data: non-indexed parameters
Next, let’s look at how to trigger events. Line 8 in the code represents the actual event triggering. Inside the fund function, it first checks that the funded amount is greater than 0.1 ETH. If there are no issues, the process continues, information is added to the array and mapping, and finally, the event is triggered. At this point, the address of the person who executed fund and the amount sent are included in the event.
...
event Funded(address indexed funder, uint256 indexed fundedAmount);
...
function fund() public payable {
require(msg.value > 100000000000000000, "value should be greater than 0.1 ETH");
s_funders.push(msg.sender);
s_addressToAmount[msg.sender] += msg.value;
emit Funded(msg.sender, msg.value); // Emitting an event
}
...
An actual example of an event on Etherscan, which includes the above-mentioned Funded event within the Funding contract, can be found here (in the following image). This example is from the log of an event that was triggered after calling the fund function following the deployment of the Funding contract.
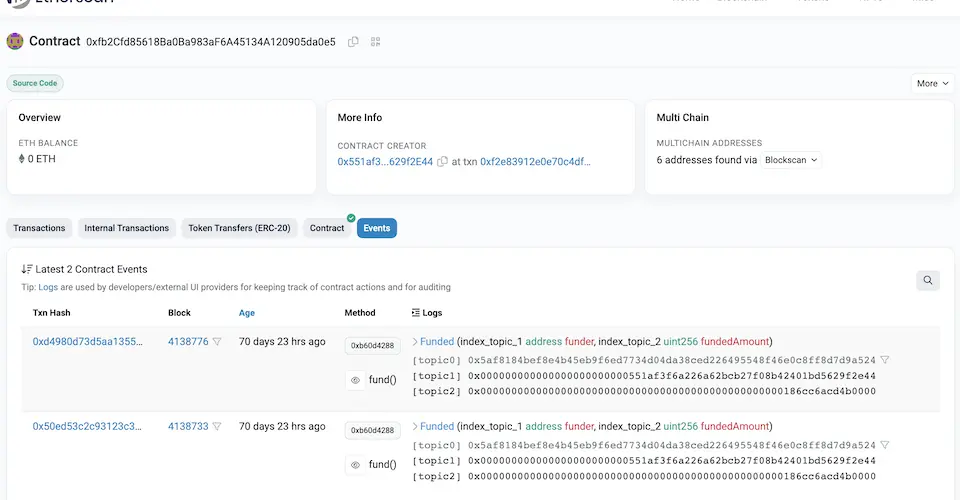
Comments